resultMap元素是MyBatis中最重要最强大的元素。他的作用是告诉MyBatis将从结果集中取出的数据转换成开发者所需要的对象。
下面是最简单的映射语句示例:
<select id="selectUserMap" resultType="map"> SELECT * FROM tb_user </select>
selectUser的<select…/>元素执行一条查询语句,查询tb_user表所有数据。resultType=“map”表示返回数据时一个Map集合(使用列名作为key,列值作为value)
示例:测试ResultMaps
1.控制器
@GetMapping("/testMap") public void testMap() { SqlSession sqlSession = FKSqlSessionFactory.getSqlSession(); //查询TB_USER表所有数据返回List集合,集合中的每个元素都是Map List<Map<String,Object>> list = sqlSession.selectList("org.fkit.mapper.UserMapper.selectUserMap"); //遍历List集合,打印每一个Map对象 list.forEach(row->System.out.println(row)); //提交事务 sqlSession.commit(); sqlSession.close(); }
控制台显示如下:
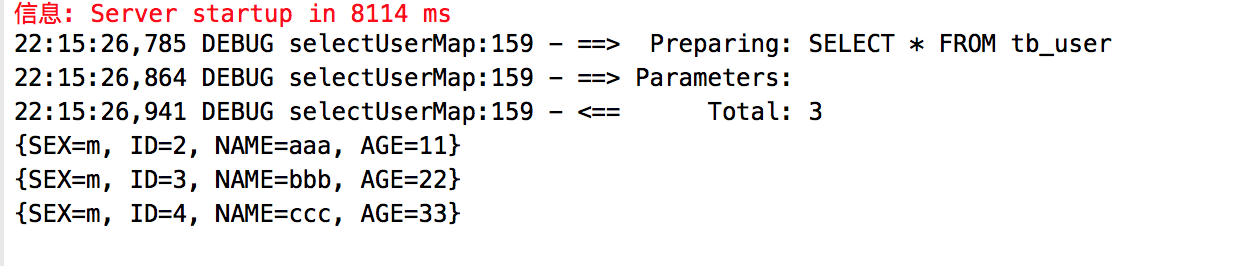
可以看到,查询语句返回的每一条数据都被封装成一个Map集合,列名作为Map集合的key,而列的值作为Map的value。
虽然数据被封装成Map集合返回,但是Map集合并不能很好的描述一个领域模型,在实际项目开发中更加建议使用JavaBean或POJO作为领域模型描述数据.
示例:将数据封装成User
1.Mapper
<resultMap id="userResultMap" type="org.fkit.domain.User"> <id property="id" column="ID" /> <result property="name" column="NAME" /> <result property="sex" column="SEX" /> <result property="age" column="AGE" /> </resultMap> <select id="selectUserMap" resultMap="userResultMap"> SELECT * FROM tb_user </select>
2.控制器
@GetMapping("/testMap") public void testMap() { SqlSession sqlSession = FKSqlSessionFactory.getSqlSession(); //查询TB_USER表所有数据返回List集合,集合中的每个元素都是Map List<User> list = sqlSession.selectList("org.fkit.mapper.UserMapper.selectUserMap"); //遍历List集合,打印每一个Map对象 list.forEach(row->System.out.println(row)); //提交事务 sqlSession.commit(); sqlSession.close(); }
在实际的项目开发中,还有更加复杂的情况,例如执行的是一个多表语句,而返回的对象关联到另一个家对象,此时简单的映射已经无法解决问题,必须使用<resultMap…/>元素完成关联映射
示例:多表映射
student表:
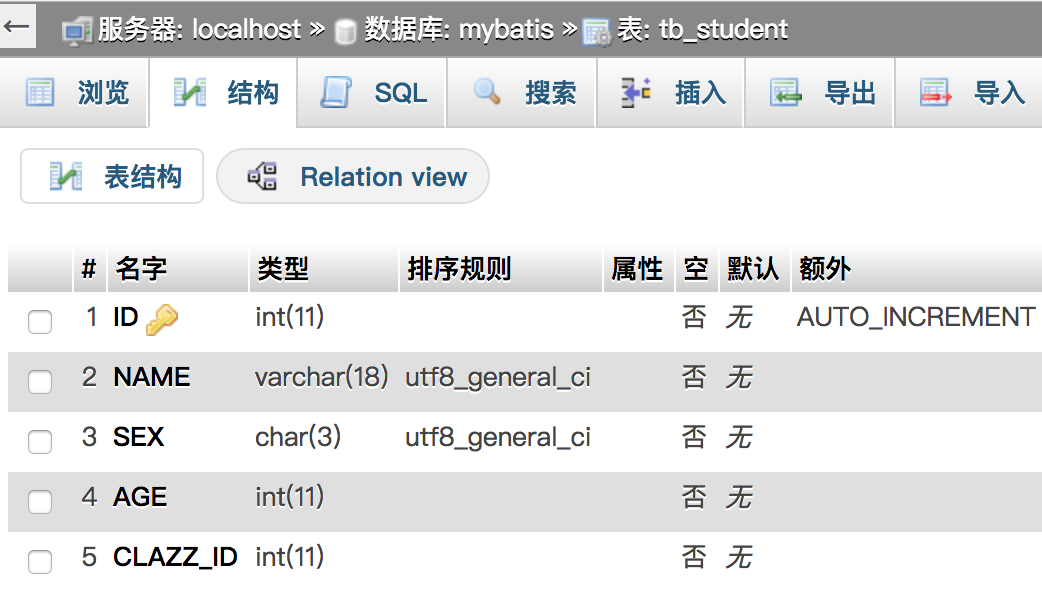
clazz表:
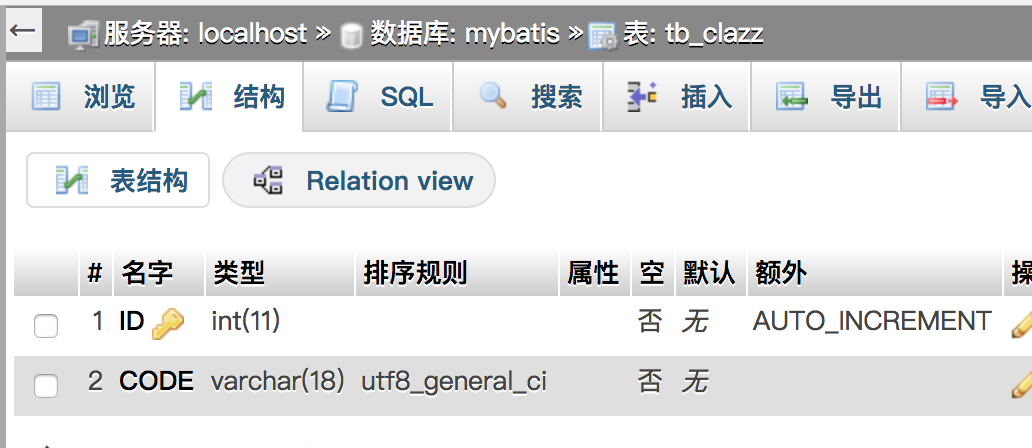
1.模型
public class Clazz implements Serializable { private Integer id; private String code; //无参数构造器 public Clazz() { super(); } … public class Student implements Serializable { private Integer id; private String name; private String sex; private Integer age; private Clazz clazz; //无参数构造器 public Student() { super(); } …
2.UserMapper
<!-- 映射学生对象的resultMap --> <resultMap id="studentResultMap" type="org.fkit.domain.Student"> <id property="id" column="ID" /> <result property="name" column="NAME" /> <result property="sex" column="SEX" /> <result property="age" column="AGE" /> <!-- 关联映射 --> <association property="clazz" column="CLAZZ_ID" javaType="org.fkit.domain.Clazz" select="selectClazzWithId" /> </resultMap> <!-- 根据班级ID查询班级 --> <select id="selectClazzWithId" resultType="org.fkit.domain.Clazz"> SELECT * FROM tb_clazz WHERE ID=#{id} </select> <!-- 查询所有学生信息 --> <select id="selecStudent" resultMap="studentResultMap"> SELECT * FROM tb_student </select>
3.控制器
@GetMapping("/testStudent") public void testStudent() { SqlSession sqlSession = FKSqlSessionFactory.getSqlSession(); List<Student> studentList = sqlSession.selectList("org.fkit.mapper.UserMapper.selecStudent"); studentList.forEach(stu->System.out.println(stu)); sqlSession.commit(); sqlSession.close(); }